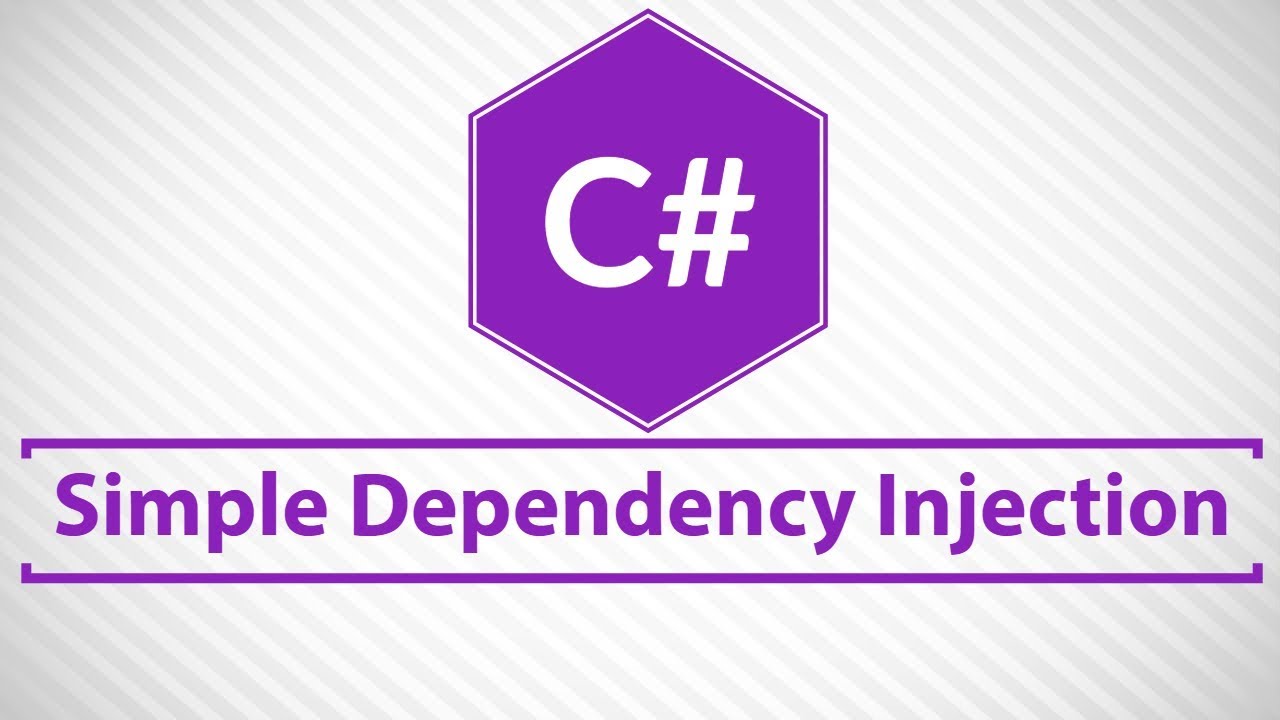
BuildServiceProvider
Check this link for Microsofts explanation of the Dependency Injection framework. You can easily use the .NET Core 6 Dependency Injection container in your (console) program. Add the package Microsoft.Extensions.DependencyInjection.
Create an instance of the ServiceCollection class and add your dependencies to this container.
# nullable disable using Microsoft.Extensions.DependencyInjection; // Create the service container var builder = new ServiceCollection() .AddSingleton<Application, Application>() .AddSingleton<IAppConfig, AppConfig>() .BuildServiceProvider(); Application app = builder.GetRequiredService<Application>(); interface IAppConfig { string Setting { get; } } class AppConfig : IAppConfig { public string Setting { get; } public AppConfig() { Console.WriteLine("AppConfig constructed"); } } class Application { IAppConfig config; public Application(IAppConfig config) { this.config = config; Console.WriteLine("Application constructed"); } }
Host.CreateDefaultBuilder
You can also make use of the Host.CreateDefaultBuilder method to create a default generic HostBuilder. This HostBuilder contains several default dependencies. Some of the more often used dependencies are shown in the picture below.

Use the code below for a minimal setup of your (console) program with the Default builder
# nullable disable using Microsoft.Extensions.DependencyInjection; using Microsoft.Extensions.Hosting; using Microsoft.Extensions.Logging; var host = CreateHostBuilder(args).Build(); Application app = host.Services.GetRequiredService<Application>(); static IHostBuilder CreateHostBuilder(string[] args) { return Host.CreateDefaultBuilder(args) .ConfigureServices( (_, services) => services .AddSingleton<Application, Application>() .AddSingleton<IAppConfig, AppConfig>()); } interface IAppConfig { string Setting { get; } } class AppConfig : IAppConfig { public string Setting { get; } public AppConfig() { Console.WriteLine("AppConfig constructed"); } } class Application { readonly IAppConfig config; public Application(IAppConfig config, ILogger<Application> logger) { this.config = config; logger.Log(LogLevel.Information, "Application constructed"); } }