To get rid of deprecated nuget packages in your solution follow the procedure below.
In Visual Studio first manage the nuget packages for your project (or solution) by right clicking the project in the solution explorer and choose Manage NuGet Packages…
Then the deprecated packages will show up in the list:

To remove those packages you have to remove them from the project file (or do an uninstall with the nuget package manager). I prefer to do this in the project file. So open the project file and remove the package entry (marked lines in the image below).
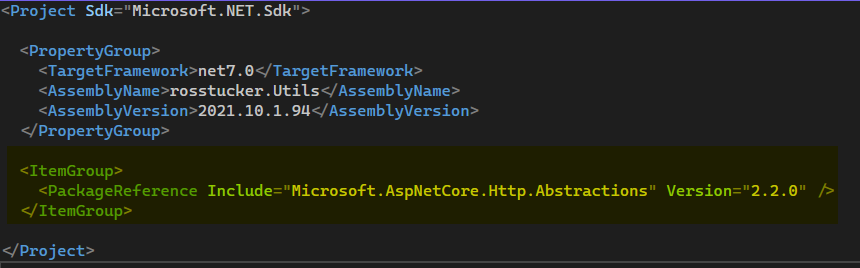
Now you have to add the FrameworkReference item to the project file. See the project file below and checkout the marked lines.
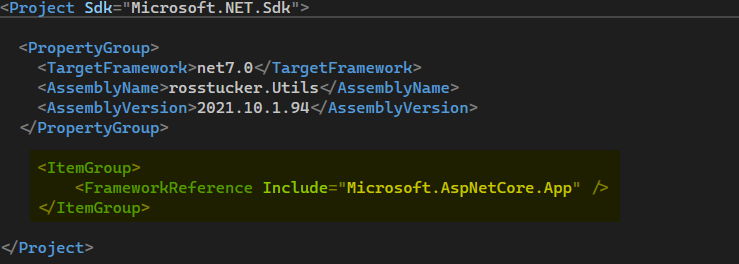
Check also this link for a more verbose explanation.