This post shows an example of scanning an image with C#. First of all start Visual Studio and create a new console application.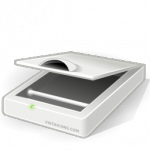
In this example I make use of the standard WIA scanning functionality in Windows 7. Reference the WIA dll in your new project. The dll can be found in the folder “c:\windows\system32\wiaaut.dll”.
The code below scans an image (A4 size) at the default 300 DPI and stores it as an uncompressed TIFF image.
namespace Scanner
{
using System;
sing System.Runtime.InteropServices;
class Program
{
const string WIA_DEVICE_PROPERTY_PAGES_ID = "3096";
const string WIA_DEVICE_PROPERTY_PAGES_ID = "3096";
const string WIA_HORIZONTAL_SCAN_RESOLUTION_DPI = "6147";
const string WIA_VERTICAL_SCAN_RESOLUTION_DPI = "6148";
const string WIA_HORIZONTAL_SCAN_START_PIXEL = "6149";
const string WIA_VERTICAL_SCAN_START_PIXEL = "6150";
const string WIA_HORIZONTAL_SCAN_SIZE_PIXELS = "6151";
const string WIA_VERTICAL_SCAN_SIZE_PIXELS = "6152";
const string WIA_SCAN_BRIGHTNESS_PERCENTS = "6154";
const string WIA_SCAN_CONTRAST_PERCENTS = "6155";
const int widthA4at300dpi = 2480;
const int heightA4at300dpi = 3508;
static void Main(string[] args)
{
WIA.CommonDialogClass commonDialogClass = new WIA.CommonDialogClass();
WIA.Device scannerDevice = null;
try
{
scannerDevice =
commonDialogClass.ShowSelectDevice(
WIA.WiaDeviceType.ScannerDeviceType,
false,
false);
SetWIAProperty(scannerDevice.Properties, WIA_DEVICE_PROPERTY_PAGES_ID, 1);
WIA.Item scannnerItem = scannerDevice.Items[1];
SetA4(scannnerItem.Properties, 300);
WIA.ImageFile scanResult =
commonDialogClass.ShowTransfer(
scannnerItem,
WIA.FormatID.wiaFormatTIFF,
false);
scanResult.SaveFile("output.tiff");
}
catch (COMException ex)
{
if ((uint)ex.ErrorCode == 0x80210015)
{
Console.WriteLine("No scanner attached");
}
else
{
Console.WriteLine("Unknown error: {0}", (uint)ex.ErrorCode);
}
}
}
private static void SetA4(WIA.IProperties properties, int dpi)
{
int width = (int)((widthA4at300dpi / 300.0) * dpi);
int height = (int)((heightA4at300dpi / 300.0) * dpi);
SetWIAProperty(properties, WIA_HORIZONTAL_SCAN_RESOLUTION_DPI, dpi);
SetWIAProperty(properties, WIA_VERTICAL_SCAN_RESOLUTION_DPI, dpi);
SetWIAProperty(properties, WIA_HORIZONTAL_SCAN_START_PIXEL, 0);
SetWIAProperty(properties, WIA_VERTICAL_SCAN_START_PIXEL, 0);
SetWIAProperty(properties, WIA_HORIZONTAL_SCAN_SIZE_PIXELS, width);
SetWIAProperty(properties, WIA_VERTICAL_SCAN_SIZE_PIXELS, height);
}
private static void SetWIAProperty(WIA.IProperties properties,
object propName, object propValue)
{
WIA.Property prop = properties.get_Item(ref propName);
prop.set_Value(ref propValue);
}
}
}